题目描述
给你一个字符串 text
,该字符串由若干被空格包围的单词组成。每个单词由一个或者多个小写英文字母组成,并且两个单词之间至少存在一个空格。题目测试用例保证 text
至少包含一个单词 。
请你重新排列空格,使每对相邻单词之间的空格数目都 相等 ,并尽可能 最大化 该数目。如果不能重新平均分配所有空格,请 将多余的空格放置在字符串末尾 ,这也意味着返回的字符串应当与原 text
字符串的长度相等。
返回 重新排列空格后的字符串 。
示例 1:
1 2 3
| 输入:text = " this is a sentence " 输出:"this is a sentence" 解释:总共有 9 个空格和 4 个单词。可以将 9 个空格平均分配到相邻单词之间,相邻单词间空格数为:9 / (4-1) = 3 个。
|
示例 2:
1 2 3
| 输入:text = " practice makes perfect" 输出:"practice makes perfect " 解释:总共有 7 个空格和 3 个单词。7 / (3-1) = 3 个空格加上 1 个多余的空格。多余的空格需要放在字符串的末尾。
|
示例 3:
1 2
| 输入:text = "hello world" 输出:"hello world"
|
示例 4:
1 2
| 输入:text = " walks udp package into bar a" 输出:"walks udp package into bar a "
|
示例 5:
提示:
1 <= text.length <= 100
text
由小写英文字母和 ' '
组成
text
中至少包含一个单词
来源:力扣(LeetCode)
题解
模拟
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| public String reorderSpaces(String text) { int n = text.length(); int spaceCount = 0; List<String> wordList = new LinkedList<>(); for (int i = 0; i < n; ) { if (text.charAt(i) == ' ') { i++; spaceCount++; continue; } int j = i; while (j < n && text.charAt(j) != ' ') j++; wordList.add(text.substring(i, j)); i = j; }
int m = wordList.size(); int divisor = spaceCount / Math.max(m - 1, 1);
String space = ""; while (divisor-- > 0) space += " ";
StringBuffer ans = new StringBuffer(); for (int i = 0; i < m; i++) { ans.append(wordList.get(i)); if (i != m - 1) ans.append(space); }
while (ans.length() != n) ans.append(" ");
return ans.toString(); }
|
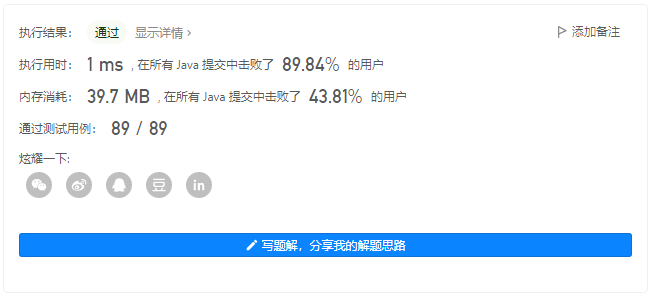
模拟(不存储单词)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| public String reorderSpaces(String text) { int n = text.length(); int wordCount = 0; int spaceCount = 0; for (int i = 0; i < n; i++) { if (text.charAt(i) == ' ') { spaceCount++; } else if (i == 0 || text.charAt(i - 1) == ' ') { wordCount++; } }
int divisor = spaceCount / Math.max(wordCount - 1, 1);
char[] ans = new char[n]; Arrays.fill(ans, ' ');
int wordIndex = 0; int ansIndex = 0;
for (int i = 0; i < wordCount; i++) { while (wordIndex < n && text.charAt(wordIndex) == ' ') wordIndex++; while (wordIndex < n && text.charAt(wordIndex) != ' ') ans[ansIndex++] = text.charAt(wordIndex++); ansIndex += divisor; }
return new String(ans); }
|
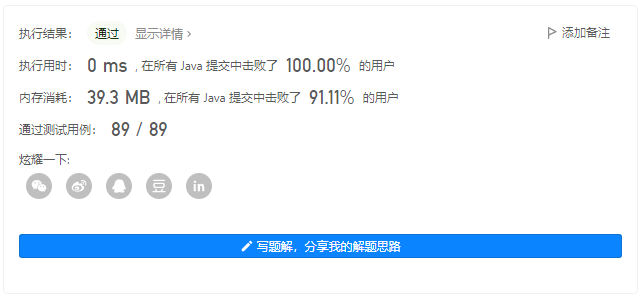
参考资料
__END__